-#include "aes.h"
+#include "mbedtls/aes.h"
#include "mifare.h"
#define MAX_CRYPTO_BLOCK_SIZE 16
@@ -71,10 +71,6 @@ enum DESFIRE_CRYPTOALGO {
struct desfire_key {
enum DESFIRE_CRYPTOALGO type;
uint8_t data[24];
- // DES_key_schedule ks1;
- // DES_key_schedule ks2;
- // DES_key_schedule ks3;
- AesCtx aes_ks;
uint8_t cmac_sk1[24];
uint8_t cmac_sk2[24];
uint8_t aes_version;
diff --git a/common/lfdemod.c b/common/lfdemod.c
index ef18b59ef..f8daec079 100644
--- a/common/lfdemod.c
+++ b/common/lfdemod.c
@@ -103,7 +103,7 @@ void computeSignalProperties(uint8_t *samples, uint32_t size) {
// we can detect noise
signalprop.isnoise = signalprop.amplitude < NOISE_AMPLITUDE_THRESHOLD;
- if (g_debugMode)
+ if (g_debugMode)
printSignal();
}
@@ -1365,8 +1365,8 @@ static int millerRawDecode(uint8_t *bits, size_t *size, int invert) {
int BiphaseRawDecode(uint8_t *bits, size_t *size, int *offset, int invert) {
//sanity check
if (*size < 51) return -1;
-
- if ( *offset < 0 ) *offset = 0;
+
+ if (*offset < 0) *offset = 0;
uint16_t bitnum = 0;
uint16_t errCnt = 0;
diff --git a/common/mbedtls/Makefile b/common/mbedtls/Makefile
index fa0dff05f..a16b2cfd9 100644
--- a/common/mbedtls/Makefile
+++ b/common/mbedtls/Makefile
@@ -59,6 +59,8 @@ CFILES = $(filter %.c, $(mbedtls_SOURCES))
CMDOBJS = $(CFILES:%.c=%.o)
CLEAN = $(CMDOBJS)
+platform = $(shell uname)
+
CC= gcc
CFLAGS= -O2 -Wall -Wno-unused-variable -Wno-unused-function
LDFLAGS= $(SYSLDFLAGS) $(mbedtls_LDFLAGS)
@@ -66,8 +68,13 @@ LIBS= $(SYSLIBS) $(MYLIBS)
DEFAULT_INCLUDES = -I. -I..
DEFS = -DHAVE_STDINT_H
+ifeq ($(platform),Darwin)
+AR= /usr/bin/ar rcs
+RANLIB= /usr/bin/ranlib
+else
AR= ar rcs
RANLIB= ranlib
+endif
RM= rm -f
TST= echo
diff --git a/common/mbedtls/config.h b/common/mbedtls/config.h
index dfda7ca40..9f4b19c5e 100644
--- a/common/mbedtls/config.h
+++ b/common/mbedtls/config.h
@@ -489,7 +489,7 @@
* This option is independent of \c MBEDTLS_AES_FEWER_TABLES.
*
*/
-//#define MBEDTLS_AES_ROM_TABLES
+#define MBEDTLS_AES_ROM_TABLES
/**
* \def MBEDTLS_AES_FEWER_TABLES
@@ -511,7 +511,7 @@
* This option is independent of \c MBEDTLS_AES_ROM_TABLES.
*
*/
-//#define MBEDTLS_AES_FEWER_TABLES
+#define MBEDTLS_AES_FEWER_TABLES
/**
* \def MBEDTLS_CAMELLIA_SMALL_MEMORY
diff --git a/common/mbedtls/platform_util.c b/common/mbedtls/platform_util.c
index a3634e246..c30a52c08 100644
--- a/common/mbedtls/platform_util.c
+++ b/common/mbedtls/platform_util.c
@@ -60,9 +60,9 @@
* mbedtls_platform_zeroize() to use a suitable implementation for their
* platform and needs.
*/
-static void *(* const volatile memset_func)(void *, int, size_t) = memset;
+//static void *(* const volatile memset_func)(void *, int, size_t) = memset;
void mbedtls_platform_zeroize(void *buf, size_t len) {
- memset_func(buf, 0, len);
+ memset(buf, 0, len);
}
#endif /* MBEDTLS_PLATFORM_ZEROIZE_ALT */
diff --git a/doc/bt_manual_v10.md b/doc/bt_manual_v10.md
index 81d87acc6..032680cfa 100644
--- a/doc/bt_manual_v10.md
+++ b/doc/bt_manual_v10.md
@@ -33,9 +33,12 @@ Built-in battery can support standalone mode, off-line sniffing, off-line readin
* Unplug your Proxmark3 RDV4.0 device from any usb cable.
* Remove the plastic upper case of Proxmark3 RDV4.0 with opener.
-* Remove temporarily the antenna with a screwdriver to expose the FPC interface.
-* Turn off all power switches, insert the FPC wire into the FPC connector, and lock the FPC connector.
-* Tear off the blue film of heat conductive double-sided tape. Align the add-on to the hole positions and gently insert it into the case.
+* Remove temporarily the antenna with a H5 (Hex/Allen) screwdriver to expose the FPC interface.
+ 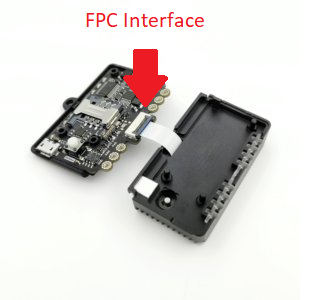
+* Turn off all power switches, insert the FPC wire into the FPC connector, and lock the FPC connector.
+ 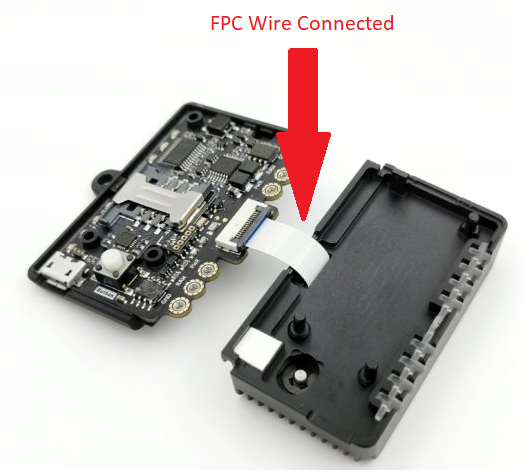
+* Tear off the blue film of heat conductive double-sided tape. Align the add-on to the hole positions and gently insert it into the case.
+ 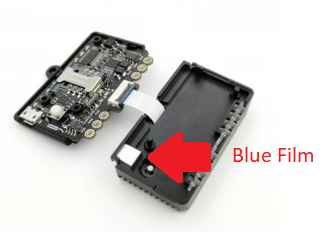
* Assembly finished!
@@ -44,6 +47,8 @@ Built-in battery can support standalone mode, off-line sniffing, off-line readin
### 4. COMPILATION / FLASHING
+#### From Source
+
Please download the latest source code from Rfid Research Group's Github repo:
https://github.com/RfidResearchGroup/proxmark3
@@ -58,6 +63,12 @@ To compile the client and firmware with FPC support, the easiest way is to
You are now ready to run the client with the serial port you got from your BT device on your laptop etc.
See instructions below.
+#### Homebrew (macOS)
+From the [homebrew-proxmark3 readme](https://github.com/RfidResearchGroup/homebrew-proxmark3)
+
+1. `brew tap rfidresearchgroup/proxmark3`
+2. `brew install --with-blueshark proxmark3`
+
### 5. CONNECT WITH BLUETOOTH
You can have both USB cable connect and BT active at the same time and connect to either serial port.
@@ -84,7 +95,8 @@ You can also switch serial port from inside the proxmark3 client using the new c
* Install driver:
http://www.silabs.com/products/development-tools/software/usb-to-uart-bridge-vcp-drivers
* Insert the adapter into the USB port. The adapter will search automatically and establish the connection. The adapter will remember the device that was first connected and after that the same device will be connected.
-* The adapter button can be used to delete memory so that other add-on can be searched and connected.
+* The adapter button can be used to delete memory so that other add-on can be searched and connected.
+
* After the connection is established, the blue state LED on add-on will turn on solid.
* look for _CP2104 USB tp UART bridge controller_ under devices in order to get the assigned serial port
@@ -122,6 +134,21 @@ The first time, your OS will ask you for pairing. The default PIN is
1234. If PIN is not typed in quickly, the client might timeout. Simply
restart it again after pairing.
+If your OS doesn't prompt you for pairing, you can do it in command line, e.g. (again, replace with your addon MAC address):
+
+```sh
+bluetoothctl
+[bluetooth]# pairable on
+[bluetooth]# scan on
+Discovery started
+...
+[CHG] Device aa:bb:cc:dd:ee:ff Name: PM3_RDV4.0
+[bluetooth]# trust aa:bb:cc:dd:ee:ff
+[bluetooth]# pair aa:bb:cc:dd:ee:ff
+[agent] Enter PIN code: 1234
+[bluetooth]# quit
+```
+
#### (2) Fast connection using dedicated USB Bluetooth adapter under Linux
@@ -133,7 +160,9 @@ the device that was first connected and after that the same device will
be connected.
2. The adapter button can be used to delete memory so that other add-on
-can be searched and connected.
+can be searched and connected.
+
+
3. After the connection is established, the blue state LED on add-on will
turn on solid.
@@ -143,6 +172,29 @@ turn on solid.
./proxmark /dev/ttyUSB0
```
+#### MacOS
+
+#### (1) Connecting rdv4.0 with Bluetooth on MacOS
+
+With MacOS Mojave 10.14.5 you could experience some continuosly disconnecting and difficult to recconnect the device at the system wakeup, to avoid this problem do a PRAM reset before to add your Proxmark3 RDV4 Blue Shark:
+
+ 1. Open a Terminal and execute this command: sudo rm -R /Library/Preferences/com.apple.Bluetooth.plist.
+ 2. Shut down your Mac.
+ 3. Then turn it on and immediately press and hold these four keys together: Option, Command, P, and R.
+ 4. You can release the keys after about 20 seconds, during which your Mac might appear to restart.
+
+After reboot you can go ahead to pairing your Proxmark3 RDV4 Blue Shark:
+
+ 5. Make sure the Blue Shark BT switch is turned ON
+ 6. On your Mac, choose Apple menu -> System Preferences, then click Bluetooth.
+ 7. Select the device in the list, then click Connect and add the 1234 pwd.
+ 8. A serial port like `/dev/tty.PM3_RDV40-DevB` will be created, use Proxmark3 client on it
+
+```sh
+./proxmark /dev/tty.PM3_RDV40-DevB
+```
+
+
### 6. OTHER NOTES
#### (1) UART and LED behavior
diff --git a/doc/md/Installation_Instructions/Linux-Installation-Instructions.md b/doc/md/Installation_Instructions/Linux-Installation-Instructions.md
index 5eb8bc66b..bb5afb268 100644
--- a/doc/md/Installation_Instructions/Linux-Installation-Instructions.md
+++ b/doc/md/Installation_Instructions/Linux-Installation-Instructions.md
@@ -13,7 +13,7 @@ You might want to follow one of these external resources to get an overview, but
# Install the required dependencies
-## On Debian / Ubuntu / Kali / ParrotOS
+## On Debian / Ubuntu / Kali / ParrotOS / Raspbian
First what we want to do is get an update for the system. If you need to upgrade do this **before** the install. An upgrade was carried out prior to following these instructions.
@@ -25,7 +25,7 @@ Install the requirements
```sh
sudo apt-get install p7zip git ca-certificates build-essential libreadline5 libreadline-dev \
-libusb-0.1-4 libusb-dev libqt4-dev perl pkg-config wget libncurses5-dev gcc-arm-none-eabi libstdc++-arm-none-eabi-newlib
+libusb-0.1-4 libusb-dev perl pkg-config wget libncurses5-dev gcc-arm-none-eabi libstdc++-arm-none-eabi-newlib libqt4-dev
```
If you don't need the graphical components of the Proxmark3 client, you can skip the installation of `libqt4-dev`.
@@ -41,8 +41,10 @@ Additional AUR packages:
```sh
yaourt -S termcap
```
-
-Note that with only these requirements, you will not get the graphical components of the Proxmark3 client. (Untested: how to get it? `yaourt -S qt4` ?)
+If you want graphical output (such as in `hw tune`):
+```sh
+sudo pacman -Su qt5-base
+```
# Clone the RRG/Iceman repository
diff --git a/doc/md/Installation_Instructions/Windows-Installation-Instructions.md b/doc/md/Installation_Instructions/Windows-Installation-Instructions.md
index ac88906da..db9a9084f 100644
--- a/doc/md/Installation_Instructions/Windows-Installation-Instructions.md
+++ b/doc/md/Installation_Instructions/Windows-Installation-Instructions.md
@@ -1,60 +1,169 @@
-# Building on Windows
-You will need to use the Gator96100 Proxspace package to assist in your windows installation.
-This can be downloaded from https://github.com/Gator96100/ProxSpace/
+# Installing on Windows
+
+There are two ways to install, build and use Proxmark3 on Windows:
+
+* Using Gator96100 **ProxSpace**, a package to assist in your Windows installation of MinGW
+* Using native **WSL**, if you're running a Windows 10 version recent enough (FCU 1709 or later)
---
-# Video Installation guide
+
+# Installing on Windows with ProxSpace
+
+## Video Installation guide
[](https://youtu.be/zzF0NCMJnYU "Windows Installation Tutorial")
-## Manual Installation
+## Driver Installation
-### Driver Installation
+Install required drivers for your Windows installation. You may need admin privileges to do this.
+Step by step guides are online such as [RiscCorps](https://store.ryscc.com/blogs/news/how-to-install-a-proxmark3-driver-on-windows-10).
-Install required drivers for your windows installation. You will may need admin privileges to do this.
-(This is covered in the video) Step by step guides are online such as [RiscCorps](https://store.ryscc.com/blogs/news/how-to-install-a-proxmark3-driver-on-windows-10)
+## Download / clone ProxSpace repo
-### Install Github
+Download the Gator96100 ProxSpace package from https://github.com/Gator96100/ProxSpace/
-Install Github for Windows https://desktop.github.com/
+If you prefer, you can clone it, provided that you installed Github for Windows https://desktop.github.com/.
-### Download / clone Proxspace repo
-
-Download the required proxspace repo. https://github.com/Gator96100/ProxSpace/
-
-Extract 'ProxSpace' to a location on drive without spaces.
+Extract 'ProxSpace' to a location path without spaces.
For example D:\OneDrive\Documents\GitHub is ok whereas C:\My Documents\My Projects\proxspace is not.
-### Clone the RRG/Iceman repository
+If you're running Windows in a Virtualbox guest, make sure not to install ProxSpace on a vbox shared drive. (It's ok later to move the `/pm3` subfolder to a shared drive and edit the `*.bat`)
-```sh
-git clone https://github.com/RfidResearchGroup/proxmark3.git
-```
-
-### Copy files to Proxspace
-
-Copy all the contents from the `proxmark3` folder into the proxspace `pm3` folder
-
-### Run the .bat
+## Launch ProxSpace
Run `runme.bat` or `runme64.bat` depending on your Windows architecture.
-Please note you will need to use `/` as you are using BASH.
+You'll get a Bash prompt and your home directory should become the ProxSpace `pm3` sub-directory.
-### Compile and use the project
+Please note you will need to use `/` in paths as you are using Bash.
+
+## Clone the RRG/Iceman repository
+
+```sh
+cd
+git clone https://github.com/RfidResearchGroup/proxmark3.git
+cd proxmark3
+```
+
+If you're a contributing developer, you probably want to be able to use `make style`. If so, you've to install astyle:
+
+```sh
+pacman -S mingw-w64-x86_64-astyle
+```
+
+## Compile and use the project
Now you're ready to follow the [compilation instructions](/doc/md/Use_of_Proxmark/0_Compilation-Instructions.md).
-The only differences are that executables end with `.exe` (e.g. `client/flasher.exe`) and that the Proxmark3 port is one of your `comX` ports where "X" is the com port number assigned to proxmark3 under Windows.
+To use the compiled client and flasher, the only differences are that executables end with `.exe` (e.g. `client/flasher.exe`) and that the Proxmark3 port is one of your `comX` ports where "X" is the com port number assigned to proxmark3 under Windows.
-So flashing will resemble
+To flash: In principle, the helper script `flash-all.sh` should auto-detect your COM port, so you can just try:
+
+```sh
+./flash-all.sh
+```
+
+If COM port detection failed, you'll have to call the flasher manually and specify the correct port:
```sh
client/flasher.exe comX -b bootrom/obj/bootrom.elf armsrc/obj/fullimage.elf
```
-And running the client will resemble
+Similarly, to run the client, you may try:
```sh
-cd client
-./proxmark3.exe comX
+./proxmark3.sh
```
+
+Or, by specifying the COM port manually:
+
+```sh
+client/proxmark3.exe comX
+```
+
+# Installing on Windows with WSL
+
+It requires to run a Windows 10 version 1709 or above. Previous versions didn't have support for COM ports.
+
+Install WSL with e.g. the standard Ubuntu.
+
+For WSL configuration, see [Manage and configure Windows Subsystem for Linux](https://docs.microsoft.com/en-us/windows/wsl/wsl-config).
+
+## X Server Installation
+
+If you want to run the graphical components of the Proxmark3 client, you need to install a X Server such as [VcXsrv](https://sourceforge.net/projects/vcxsrv/) or [Xming](https://sourceforge.net/projects/xming/) and launch it, e.g. by executing XLaunch.
+
+## Dependencies
+
+Enter WSL prompt (`wsl`) and from there, follow the [Linux Installation Instructions](/doc/md/Installation_Instructions/Linux-Installation-Instructions.md) for Ubuntu, summarized here below:
+
+```sh
+sudo apt-get update
+sudo apt-get install p7zip git ca-certificates build-essential libreadline5 libreadline-dev libusb-0.1-4 \
+libusb-dev perl pkg-config wget libncurses5-dev gcc-arm-none-eabi libstdc++-arm-none-eabi-newlib \
+libqt4-dev
+```
+
+If you don't need the graphical components of the Proxmark3 client, you can skip the installation of `libqt4-dev`.
+
+## Clone the RRG/Iceman repository
+
+```sh
+git clone https://github.com/RfidResearchGroup/proxmark3.git
+```
+
+## Compile and use the project
+
+Now you're ready to follow the [compilation instructions](/doc/md/Use_of_Proxmark/0_Compilation-Instructions.md).
+
+To use the compiled client and flasher, the only difference is that the Proxmark3 port is translated from your `comX` port where "X" is the com port number assigned to proxmark3 under Windows, to a `/dev/ttySX`.
+
+Depending on the Windows version, you might need to give permission to the current user to access `/dev/ttySX`: (change X to your port number)
+
+```sh
+ls -al /dev/ttySX
+groups|grep dialout
+```
+
+If group ownership is `dialout` and your user is member of `dialout` group, all is fine. Else you'll have to provide access to `/dev/ttySX`: (Unfortunately the access rights of the port won't survive and will have to be fixed again next time.)
+
+```sh
+sudo chmod 666 /dev/ttySX
+```
+
+If you installed a X Server and compiled the Proxmark3 with QT4 support, you've to export the `DISPLAY` environment variable:
+
+```sh
+export DISPLAY=:0
+```
+
+and add it to your Bash profile for the next times:
+
+```sh
+echo "export DISPLAY=:0" >> ~/.bashrc
+```
+
+To flash: In principle, the helper script `flash-all.sh` should auto-detect your COMX==/dev/ttySX port, so you can just try:
+
+```sh
+./flash-all.sh
+```
+
+If port detection failed, you'll have to call the flasher manually and specify the correct port:
+
+```sh
+client/flasher /dev/ttySX -b bootrom/obj/bootrom.elf armsrc/obj/fullimage.elf
+```
+
+Similarly, to run the client, you may try:
+
+```sh
+./proxmark3.sh
+```
+
+Or, by specifying the COM port manually:
+
+```sh
+client/proxmark3 /dev/ttySX
+```
+
+Note that it may take a quite long time for a freshly plugged Proxmark3 to be visible on a WSL /dev/ttySX port.
diff --git a/doc/md/Use_of_Proxmark/0_Compilation-Instructions.md b/doc/md/Use_of_Proxmark/0_Compilation-Instructions.md
index ffe312aac..7493fba1a 100644
--- a/doc/md/Use_of_Proxmark/0_Compilation-Instructions.md
+++ b/doc/md/Use_of_Proxmark/0_Compilation-Instructions.md
@@ -1,5 +1,16 @@
# Compilation instructions
+## Tuning compilation parameters
+
+The client and the Proxmark3 firmware should always be in sync.
+Nevertheless, the firmware can be tuned depending on the Proxmark3 platform and options.
+
+Indeed, the RRG/Iceman fork can be used on other Proxmark3 hardware platforms as well.
+
+Via some definitions, you can adjust the firmware for a given platform, but also to add features like the support of the Blue Shark add-on or to select which standalone mode to embed.
+
+To learn how to adjust the firmware, please read [Advanced compilation parameters](/doc/md/Use_of_Proxmark/4_Advanced-compilation-parameters.md).
+
## Get the latest commits
```sh
@@ -15,12 +26,22 @@ make clean && make all
## Flash the BOOTROM & FULLIMAGE
+In most cases, you can run the script `flash-all.sh` which try to auto-detect the port to use, on several OS.
+
+For the other cases, specify the port by yourself. For example, for a Proxmark3 connected via USB under Linux:
+
```sh
client/flasher /dev/ttyACM0 -b bootrom/obj/bootrom.elf armsrc/obj/fullimage.elf
```
## Run the client
+In most cases, you can run the script `proxmark3.sh` which try to auto-detect the port to use, on several OS.
+
+For the other cases, specify the port by yourself. For example, for a Proxmark3 connected via USB under Linux:
+
+Here, for example, for a Proxmark3 connected via USB under Linux:
+
```sh
cd client
./proxmark3 /dev/ttyACM0
diff --git a/doc/md/Use_of_Proxmark/4_Advanced-compilation-parameters.md b/doc/md/Use_of_Proxmark/4_Advanced-compilation-parameters.md
new file mode 100644
index 000000000..aea5b5e30
--- /dev/null
+++ b/doc/md/Use_of_Proxmark/4_Advanced-compilation-parameters.md
@@ -0,0 +1,82 @@
+# Advanced compilation parameters
+
+The client and the Proxmark3 firmware should always be in sync.
+Nevertheless, the firmware can be tuned depending on the Proxmark3 platform and options.
+
+Indeed, the RRG/Iceman fork can be used on other Proxmark3 hardware platforms as well.
+
+Via some definitions, you can adjust the firmware for a given platform, but also to add features like the support of the Blue Shark add-on or to select which standalone mode to embed.
+
+## Client
+
+The client doesn't depend on the capabilities of the Proxmark3 it's connected to.
+So you can use the same client for different Proxmark3 platforms, given that everything is running the same version.
+
+## Firmware
+
+By default, the firmware is of course tuned for the Proxmark3 Rdv4.0 device, which has built-in support for 256kb onboard flash SPI memory, Sim module (smart card support), FPC connector.
+These features make it very different from all other devices, there is non other like this one.
+
+**Recommendation**: if you don't have a RDV4, we strongly recommend your device to have at least a 512kb arm chip, since this repo is on the very edge of 256kb limit.
+
+A firmware built for the RDV4 can still run on the other platforms as it will auto-detect during boot that external SPI and Sim are not present, still it will boot faster if it's tuned to the platform.
+
+If you need to tune things and save the configuration, create a file `Makefile.platform` in the root directory of the repository, see `Makefile.platform.sample`.
+For an up-to-date exhaustive list of options, you can run `make PLATFORM=`.
+
+## PLATFORM
+
+Here are the supported values you can assign to `PLATFORM` in `Makefile.platform`:
+
+| PLATFORM | DESCRIPTION |
+|-----------------|----------------------------------------|
+| PM3RDV4 (def) | Proxmark3 rdv4 with AT91SAM7S512 |
+| PM3EVO | Proxmark3 EVO with AT91SAM7S512 |
+| PM3EASY | Proxmark3 rdv3 Easy with AT91SAM7S256 |
+| PM3RDV2 | Proxmark3 rdv2 with AT91SAM7S512 |
+| PM3OLD256 | Proxmark3 V1 with AT91SAM7S256 |
+| PM3OLD512 | Proxmark3 V1 with AT91SAM7S512 |
+
+By default `PLATFORM=PM3RDV4`.
+
+Known issues:
+
+* 256kb Arm chip devices: The compiled firmware image from this repo may/will be too large for your device.
+* PM3 Evo: it has a different led/button pin assignment. It tends to be messed up.
+
+## PLATFORM_EXTRAS
+
+Here are the supported values you can assign to `PLATFORM_EXTRAS` in `Makefile.platform`:
+
+| PLATFORM_EXTRAS | DESCRIPTION |
+|-----------------|----------------------------------------|
+| BTADDON | Proxmark3 rdv4 BT add-on |
+
+By default `PLATFORM_EXTRAS=`.
+
+If you have installed a Blue Shark add-on on your RDV4, define `PLATFORM_EXTRAS=BTADDON` in your `Makefile.platform`.
+
+
+## STANDALONE
+
+The RRG/Iceman fork gives you to easily choose which standalone mode to embed in the firmware.
+
+Here are the supported values you can assign to `STANDALONE` in `Makefile.platform`:
+
+| STANDALONE | DESCRIPTION |
+|-----------------|----------------------------------------|
+| | No standalone mode
+| LF_SAMYRUN (def)| HID26 read/clone/sim - Samy Kamkar
+| LF_ICERUN | standalone mode skeleton - iceman
+| LF_PROXBRUTE | HID ProxII bruteforce - Brad Antoniewicz
+| LF_HIDBRUTE | HID corporate 1000 bruteforce - Federico dotta & Maurizio Agazzini
+| HF_YOUNG | Mifare sniff/simulation - Craig Young
+| HF_MATTYRUN | Mifare sniff/clone - Matías A. Ré Medina
+| HF_COLIN | Mifare ultra fast sniff/sim/clone - Colin Brigato
+| HF_BOG | 14a sniff with ULC/ULEV1/NTAG auth storing in flashmem - Bogito
+
+By default `STANDALONE=LF_SAMYRUN`.
+
+## Next step
+
+See [Compilation instructions](/doc/md/Use_of_Proxmark/0_Compilation-Instructions.md)
diff --git a/doc/new_frame_format.md b/doc/new_frame_format.md
index 76424f8b6..17c94120a 100644
--- a/doc/new_frame_format.md
+++ b/doc/new_frame_format.md
@@ -373,9 +373,9 @@ It was needed to tune pm3 RX usart `maxtry` :
time client/proxmark3 -p /dev/ttyUSB0 -b 115200 -c "lf read"
6.28s
- time client/proxmark3 -p /dev/ttyACM0 -c "mem save f foo_usb"
+ time client/proxmark3 -p /dev/ttyACM0 -c "mem dump f foo_usb"
1.48s
- time client/proxmark3 -p /dev/ttyUSB0 -b 115200 -c "mem save f foo_fpc"
+ time client/proxmark3 -p /dev/ttyUSB0 -b 115200 -c "mem dump f foo_fpc"
25.34s
diff --git a/doc/termux_notes.md b/doc/termux_notes.md
new file mode 100644
index 000000000..f568c6f16
--- /dev/null
+++ b/doc/termux_notes.md
@@ -0,0 +1,68 @@
+# Proxmark 3 on Android
+
+## Requirements
+- Android phone
+- Kernel with one of:
+ - USB_ACM driver
+ - module loading enabled
+ - published sources
+- Root
+- [Termux](https://play.google.com/store/apps/details?id=com.termux)
+
+## Notes
+From official Proxmark3 wiki:
+ > In any case, you would need a USB-C to A or USB-OTG cable to connect Proxmark3 to your Android device. Some Android devices may not supply enough power (USB-OTG = 100mA), and need a USB Y-cable and external battery, otherwise they will get strange failures.
+ref : https://github.com/Proxmark/proxmark3/wiki/android
+
+## Tested setup
+- OnePlus 5 (arm64, USB-C)
+- [OmniROM (Android 9)](https://www.omnirom.org/)
+- [OmniROM kernel](https://www.omnirom.org/)
+- [Magisk 19.3](https://github.com/topjohnwu/Magisk/)
+
+## Setup
+### Getting ncurses with termcap
+You need `termcap` to build the Proxmark3 client. Unfortunately, the prebuilt `ncurses` package does not include it. Since [this PR](https://github.com/termux/termux-packages/pull/2131) hasn't been merged yet, you will have to build `ncurses` yourself
+
+1. Clone the [termux-packages](https://github.com/termux/termux-packages) repo
+2. Apply [the PR](https://github.com/termux/termux-packages/pull/2131)
+3. Build `ncurses`. Refer to the [build docs](https://github.com/termux/termux-packages/blob/master/docs/BUILD_ENVIRONMENT.md) for more information. The easiest way is to use Docker:
+ - `./scripts/run-docker.sh`
+ - `./build-package.sh ncurses`
+ - the built packages will be under the `debs` directory
+4. Copy `ncurses_6.1.x-x_aarch64.deb` to your phone's `/sdcard`
+### Setting up Termux
+1. Install [Termux](https://play.google.com/store/apps/details?id=com.termux) and start it
+2. Run the following commands:
+```
+pkg install make, clang, clang++, readline-dev, libclang-dev, libc++, git, tsu
+termux-setup-storage
+dpkg -i /sdcard/ncurses_6.1.x-x_aarch64.deb
+git clone https://github.com/RfidResearchGroup/proxmark3.git
+```
+### Building Proxmark3 client
+1. Edit `proxmark3/client/Makefile` and append `-fPIC` to the `CFLAGS` variable (line 30)
+2. `make clean && make client`
+
+### USB_ACM
+You need the `USB_ACM` driver enabled and working to communicate with the Proxmark3. To see if it's working, run `tsudo ls /dev/tty*` and it should list `/dev/ttyACM0` (or similar). If you see this, congratulations, skip this step!
+
+#### Enable the driver
+If your kernel has module loading enabled, you should be able to build the module separately and load it on your system without any changes. Otherwise, grab your kernel sources and edit your build config to include `CONFIG_USB_ACM=y`. On the tested kernel, this was under: `android_kernel_oneplus_msm8998/arch/arm64/configs/omni_oneplus5_defconfig`
+
+#### Building the kernel
+If using a custom kernel, refer to the build instructions provided by its maintainer. Otherwise, follow the standard Linux kernel build procedure
+
+#### Flashing the kernel
+You can flash the kernel however it suits you. On the tested device, this was achieved using [TWRP](https://twrp.me/), the most popular custom recovery
+
+### Testing
+Open Termux and start the Proxmark3 client:
+```
+tsudo proxmark3/client/proxmark3 /dev/ttyACM0
+```
+Everything should work just like if it was your PC!
+
+### Troubleshooting
+- `dmesg | grep usb` - useful debug info
+- `/proc/config.gz` - contains your kernel's build configuration. Look for `CONFIG_USB_ACM`, which should be enabled
diff --git a/flash-all.sh b/flash-all.sh
index 27e1fb526..d4fcdc975 100755
--- a/flash-all.sh
+++ b/flash-all.sh
@@ -1,4 +1,5 @@
#!/bin/bash
-cd $(dirname "$0")
+PM3PATH=$(dirname "$0")
+cd "$PM3PATH" || exit 1
. proxmark3.sh
diff --git a/flash-bootrom.sh b/flash-bootrom.sh
index 27e1fb526..d4fcdc975 100755
--- a/flash-bootrom.sh
+++ b/flash-bootrom.sh
@@ -1,4 +1,5 @@
#!/bin/bash
-cd $(dirname "$0")
+PM3PATH=$(dirname "$0")
+cd "$PM3PATH" || exit 1
. proxmark3.sh
diff --git a/flash-fullimage.sh b/flash-fullimage.sh
index 27e1fb526..d4fcdc975 100755
--- a/flash-fullimage.sh
+++ b/flash-fullimage.sh
@@ -1,4 +1,5 @@
#!/bin/bash
-cd $(dirname "$0")
+PM3PATH=$(dirname "$0")
+cd "$PM3PATH" || exit 1
. proxmark3.sh
diff --git a/include/common.h b/include/common.h
index 3dbf77622..dec2261ec 100644
--- a/include/common.h
+++ b/include/common.h
@@ -19,9 +19,20 @@ extern "C" {
#include
#include
#include
-#include
+
typedef unsigned char byte_t;
+#ifdef _MSC_VER
+typedef DWORD uint32_t;
+typedef BYTE uint8_t;
+#define PACKED
+// stuff
+#else
+#include
+#include
+#define PACKED __attribute__((packed))
+#endif
+
// debug
#define DBG_NONE 0 // no messages
#define DBG_ERROR 1 // errors only
@@ -30,9 +41,6 @@ typedef unsigned char byte_t;
#define DBG_EXTENDED 4 // errors + info + debug + breaking debug messages
extern int DBGLEVEL;
-// Flashmem spi baudrate
-extern uint32_t FLASHMEM_SPIBAUDRATE;
-
// reader voltage field detector
#define MF_MINFIELDV 4000
@@ -49,74 +57,87 @@ extern uint32_t FLASHMEM_SPIBAUDRATE;
#endif
#define RAMFUNC __attribute((long_call, section(".ramfunc")))
-// RDV40 Section
-// 256kb divided into 4k sectors.
-//
-// 0x3F000 - 1 4kb sector = signature
-// 0x3E000 - 1 4kb sector = settings
-// 0x3D000 - 1 4kb sector = default T55XX keys dictionary
-// 0x3B000 - 1 4kb sector = default ICLASS keys dictionary
-// 0x39000 - 2 4kb sectors = default MFC keys dictionary
-//
-#ifndef FLASH_MEM_BLOCK_SIZE
-# define FLASH_MEM_BLOCK_SIZE 256
+#ifndef ROTR
+# define ROTR(x,n) (((uintmax_t)(x) >> (n)) | ((uintmax_t)(x) << ((sizeof(x) * 8) - (n))))
#endif
-#ifndef FLASH_MEM_MAX_SIZE
-# define FLASH_MEM_MAX_SIZE 0x40000 // (262144)
+#ifndef ROTL
+# define ROTL(x,n) (((uintmax_t)(x) << (n)) | ((uintmax_t)(x) >> ((sizeof(x) * 8) - (n))))
#endif
-#ifndef FLASH_MEM_MAX_4K_SECTOR
-# define FLASH_MEM_MAX_4K_SECTOR 0x3F000
+// endian change for 64bit
+#ifdef __GNUC__
+#ifndef BSWAP_64
+#define BSWAP_64(x) __builtin_bswap64(x)
+#endif
+#else
+#ifdef _MSC_VER
+#ifndef BSWAP_64
+#define BSWAP_64(x) _byteswap_uint64(x)
+#endif
+#else
+#ifndef BSWAP_64
+#define BSWAP_64(x) \
+ (((uint64_t)(x) << 56) | \
+ (((uint64_t)(x) << 40) & 0xff000000000000ULL) | \
+ (((uint64_t)(x) << 24) & 0xff0000000000ULL) | \
+ (((uint64_t)(x) << 8) & 0xff00000000ULL) | \
+ (((uint64_t)(x) >> 8) & 0xff000000ULL) | \
+ (((uint64_t)(x) >> 24) & 0xff0000ULL) | \
+ (((uint64_t)(x) >> 40) & 0xff00ULL) | \
+ ((uint64_t)(x) >> 56))
+#endif
+#endif
#endif
-
-#ifndef FLASH_MEM_ID_LEN
-# define FLASH_MEM_ID_LEN 8
+// endian change for 32bit
+#ifdef __GNUC__
+#ifndef BSWAP_32
+#define BSWAP_32(x) __builtin_bswap32(x)
+#endif
+#else
+#ifdef _MSC_VER
+#ifndef BSWAP_32
+#define BSWAP_32(x) _byteswap_ulong(x)
+#endif
+#else
+#ifndef BSWAP_32
+# define BSWAP_32(x) \
+ ((((x) & 0xff000000) >> 24) | (((x) & 0x00ff0000) >> 8) | \
+ (((x) & 0x0000ff00) << 8) | (((x) & 0x000000ff) << 24))
+#endif
+#endif
#endif
-#ifndef FLASH_MEM_SIGNATURE_LEN
-# define FLASH_MEM_SIGNATURE_LEN 128
+#define EVEN 0
+#define ODD 1
+
+// Nibble logic
+#ifndef NIBBLE_HIGH
+# define NIBBLE_HIGH(b) ( (b & 0xF0) >> 4 )
#endif
-#ifndef FLASH_MEM_SIGNATURE_OFFSET
-// -1 for historical compatibility with already released Proxmark3 RDV4.0 devices
-# define FLASH_MEM_SIGNATURE_OFFSET (FLASH_MEM_MAX_SIZE - FLASH_MEM_SIGNATURE_LEN - 1)
+#ifndef NIBBLE_LOW
+# define NIBBLE_LOW(b) ( b & 0x0F )
#endif
-#ifndef T55XX_CONFIG_LEN
-# define T55XX_CONFIG_LEN sizeof( t55xx_config )
+#ifndef CRUMB
+# define CRUMB(b,p) (((b & (0x3 << p) ) >> p ) & 0xF)
#endif
-#ifndef T55XX_CONFIG_OFFSET
-# define T55XX_CONFIG_OFFSET (FLASH_MEM_MAX_4K_SECTOR - 0x2000)
+#ifndef SWAP_NIBBLE
+# define SWAP_NIBBLE(b) ( (NIBBLE_LOW(b)<< 4) | NIBBLE_HIGH(b))
#endif
-// Reserved space for T55XX PWD = 4 kb
-#ifndef DEFAULT_T55XX_KEYS_OFFSET
-# define DEFAULT_T55XX_KEYS_OFFSET (FLASH_MEM_MAX_4K_SECTOR - 0x3000)
+// Binary Encoded Digit
+#ifndef BCD2DEC
+# define BCD2DEC(bcd) HornerScheme(bcd, 0x10, 10)
#endif
-// Reserved space for iClass keys = 4 kb
-#ifndef DEFAULT_ICLASS_KEYS_OFFSET
-# define DEFAULT_ICLASS_KEYS_OFFSET (FLASH_MEM_MAX_4K_SECTOR - 0x4000)
+#ifndef DEC2BCD
+# define DEC2BCD(dec) HornerScheme(dec, 10, 0x10)
#endif
-// Reserved space for MIFARE Keys = 8 kb
-#ifndef DEFAULT_MF_KEYS_OFFSET
-# define DEFAULT_MF_KEYS_OFFSET (FLASH_MEM_MAX_4K_SECTOR - 0x6000)
-#endif
-
-
-
-// RDV40, validation structure to help identifying that client/firmware is talking with RDV40
-typedef struct {
- uint8_t magic[4];
- uint8_t flashid[FLASH_MEM_ID_LEN];
- uint8_t signature[FLASH_MEM_SIGNATURE_LEN];
-} __attribute__((__packed__)) rdv40_validation_t;
-
-
#ifdef __cplusplus
}
#endif
diff --git a/include/hitag.h b/include/hitag.h
index 742d7ecd0..9df93fcf7 100644
--- a/include/hitag.h
+++ b/include/hitag.h
@@ -14,11 +14,7 @@
#ifndef HITAG_H__
#define HITAG_H__
-#ifdef _MSC_VER
-#define PACKED
-#else
-#define PACKED __attribute__((packed))
-#endif
+#include "common.h"
typedef enum {
RHTSF_CHALLENGE = 01,
@@ -31,6 +27,7 @@ typedef enum {
WHT2F_CRYPTO = 24,
RHT2F_TEST_AUTH_ATTEMPTS = 25,
RHT2F_UID_ONLY = 26,
+ WHT2F_PASSWORD = 27,
} hitag_function;
typedef struct {
diff --git a/include/mifare.h b/include/mifare.h
index 87ba3a74e..9d444e833 100644
--- a/include/mifare.h
+++ b/include/mifare.h
@@ -46,7 +46,7 @@ typedef struct {
uint8_t sak;
uint8_t ats_len;
uint8_t ats[256];
-} __attribute__((__packed__)) iso14a_card_select_t;
+} PACKED iso14a_card_select_t;
typedef enum ISO14A_COMMAND {
ISO14A_CONNECT = (1 << 0),
@@ -129,7 +129,7 @@ typedef struct {
typedef struct {
uint8_t atr_len;
uint8_t atr[30];
-} __attribute__((__packed__)) smart_card_atr_t;
+} PACKED smart_card_atr_t;
typedef enum SMARTCARD_COMMAND {
SC_CONNECT = (1 << 0),
@@ -167,7 +167,7 @@ typedef struct {
uint8_t iccode[2];
uint8_t mrt[6];
uint8_t servicecode[2];
-} __attribute__((__packed__)) felica_card_select_t;
+} PACKED felica_card_select_t;
typedef enum FELICA_COMMAND {
FELICA_CONNECT = (1 << 0),
diff --git a/include/pm3_cmd.h b/include/pm3_cmd.h
index 772ec02b9..d271d3099 100644
--- a/include/pm3_cmd.h
+++ b/include/pm3_cmd.h
@@ -13,20 +13,11 @@
#ifndef __PM3_CMD_H
#define __PM3_CMD_H
+#include "common.h"
+
// Use it e.g. when using slow links such as BT
#define USART_SLOW_LINK
-#ifdef _MSC_VER
-typedef DWORD uint32_t;
-typedef BYTE uint8_t;
-#define PACKED
-// stuff
-#else
-#include
-#include
-#define PACKED __attribute__((packed))
-#endif
-
#define PM3_CMD_DATA_SIZE 512
#define PM3_CMD_DATA_SIZE_MIX ( PM3_CMD_DATA_SIZE - 3 * sizeof(uint64_t) )
@@ -216,6 +207,7 @@ typedef struct {
#define CMD_FINISH_WRITE 0x0003
#define CMD_HARDWARE_RESET 0x0004
#define CMD_START_FLASH 0x0005
+#define CMD_CHIP_INFO 0x0006
#define CMD_NACK 0x00fe
#define CMD_ACK 0x00ff
@@ -235,9 +227,9 @@ typedef struct {
#define CMD_CAPABILITIES 0x0112
#define CMD_QUIT_SESSION 0x0113
#define CMD_SET_DBGMODE 0x0114
+#define CMD_STANDALONE 0x0115
// RDV40, Flash memory operations
-#define CMD_FLASHMEM_READ 0x0120
#define CMD_FLASHMEM_WRITE 0x0121
#define CMD_FLASHMEM_WIPE 0x0122
#define CMD_FLASHMEM_DOWNLOAD 0x0123
@@ -541,6 +533,9 @@ typedef struct {
/* Set if this device understands the extend start flash command */
#define DEVICE_INFO_FLAG_UNDERSTANDS_START_FLASH (1<<4)
+/* Set if this device understands the chip info command */
+#define DEVICE_INFO_FLAG_UNDERSTANDS_CHIP_INFO (1<<5)
+
/* CMD_START_FLASH may have three arguments: start of area to flash,
end of area to flash, optional magic.
The bootrom will not allow to overwrite itself unless this magic
diff --git a/include/pmflash.h b/include/pmflash.h
new file mode 100644
index 000000000..b7bf39ddc
--- /dev/null
+++ b/include/pmflash.h
@@ -0,0 +1,97 @@
+//-----------------------------------------------------------------------------
+// (c) RFID Research Group - 2019
+//
+// This code is licensed to you under the terms of the GNU GPL, version 2 or,
+// at your option, any later version. See the LICENSE.txt file for the text of
+// the license.
+//
+//-----------------------------------------------------------------------------
+// RDV4 flash constants
+//-----------------------------------------------------------------------------
+
+#ifndef __PMFLASH_H
+#define __PMFLASH_H
+
+#ifdef __cplusplus
+extern "C" {
+#endif
+
+#include
+#include
+#include
+
+#include "common.h"
+
+// Flashmem spi baudrate
+extern uint32_t FLASHMEM_SPIBAUDRATE;
+
+// RDV40 Section
+// 256kb divided into 4k sectors.
+//
+// 0x3F000 - 1 4kb sector = signature
+// 0x3E000 - 1 4kb sector = settings
+// 0x3D000 - 1 4kb sector = default T55XX keys dictionary
+// 0x3B000 - 1 4kb sector = default ICLASS keys dictionary
+// 0x39000 - 2 4kb sectors = default MFC keys dictionary
+//
+#ifndef FLASH_MEM_BLOCK_SIZE
+# define FLASH_MEM_BLOCK_SIZE 256
+#endif
+
+#ifndef FLASH_MEM_MAX_SIZE
+# define FLASH_MEM_MAX_SIZE 0x40000 // (262144)
+#endif
+
+#ifndef FLASH_MEM_MAX_4K_SECTOR
+# define FLASH_MEM_MAX_4K_SECTOR 0x3F000
+#endif
+
+
+#ifndef FLASH_MEM_ID_LEN
+# define FLASH_MEM_ID_LEN 8
+#endif
+
+#ifndef FLASH_MEM_SIGNATURE_LEN
+# define FLASH_MEM_SIGNATURE_LEN 128
+#endif
+
+#ifndef FLASH_MEM_SIGNATURE_OFFSET
+// -1 for historical compatibility with already released Proxmark3 RDV4.0 devices
+# define FLASH_MEM_SIGNATURE_OFFSET (FLASH_MEM_MAX_SIZE - FLASH_MEM_SIGNATURE_LEN - 1)
+#endif
+
+#ifndef T55XX_CONFIG_LEN
+# define T55XX_CONFIG_LEN sizeof( t55xx_config )
+#endif
+
+#ifndef T55XX_CONFIG_OFFSET
+# define T55XX_CONFIG_OFFSET (FLASH_MEM_MAX_4K_SECTOR - 0x2000)
+#endif
+
+// Reserved space for T55XX PWD = 4 kb
+#ifndef DEFAULT_T55XX_KEYS_OFFSET
+# define DEFAULT_T55XX_KEYS_OFFSET (FLASH_MEM_MAX_4K_SECTOR - 0x3000)
+#endif
+
+// Reserved space for iClass keys = 4 kb
+#ifndef DEFAULT_ICLASS_KEYS_OFFSET
+# define DEFAULT_ICLASS_KEYS_OFFSET (FLASH_MEM_MAX_4K_SECTOR - 0x4000)
+#endif
+
+// Reserved space for MIFARE Keys = 8 kb
+#ifndef DEFAULT_MF_KEYS_OFFSET
+# define DEFAULT_MF_KEYS_OFFSET (FLASH_MEM_MAX_4K_SECTOR - 0x6000)
+#endif
+
+// RDV40, validation structure to help identifying that client/firmware is talking with RDV40
+typedef struct {
+ uint8_t magic[4];
+ uint8_t flashid[FLASH_MEM_ID_LEN];
+ uint8_t signature[FLASH_MEM_SIGNATURE_LEN];
+} PACKED rdv40_validation_t;
+
+#ifdef __cplusplus
+}
+#endif
+
+#endif // __PMFLASH_H
diff --git a/include/proxmark3.h b/include/proxmark3.h
index 8748e82d8..469713b5b 100644
--- a/include/proxmark3.h
+++ b/include/proxmark3.h
@@ -15,6 +15,7 @@
#include "at91sam7s512.h"
#include "config_gpio.h"
#include "pm3_cmd.h"
+#include "common.h"
#define WDT_HIT() AT91C_BASE_WDTC->WDTC_WDCR = 0xa5000001
@@ -71,8 +72,6 @@
#define COTAG_BITS 264
#endif
-//#define PACKED __attribute__((__packed__))
-
#define LED_A_ON() HIGH(GPIO_LED_A)
#define LED_A_OFF() LOW(GPIO_LED_A)
#define LED_A_INV() INVBIT(GPIO_LED_A)
@@ -116,7 +115,7 @@ struct version_information {
char clean; /* 1: Tree was clean, no local changes. 0: Tree was unclean. 2: Couldn't be determined */
char gitversion[50]; /* String with the git revision */
char buildtime[30]; /* string with the build time */
-} __attribute__((packed));
+} PACKED;
#define COMMON_AREA_MAGIC 0x43334d50 // "PM3C"
#define COMMON_AREA_COMMAND_NONE 0
@@ -129,8 +128,8 @@ struct common_area {
unsigned int bootrom_present: 1; /* Set when a bootrom that is capable of parsing the common area is present */
unsigned int osimage_present: 1; /* Set when a osimage that is capable of parsing the common area is present */
unsigned int button_pressed: 1;
- } __attribute__((packed)) flags;
+ } PACKED flags;
int arg1, arg2;
-} __attribute__((packed));
+} PACKED;
#endif
diff --git a/liblua/Makefile b/liblua/Makefile
index bf2a4fa3c..2b3d7614f 100644
--- a/liblua/Makefile
+++ b/liblua/Makefile
@@ -12,13 +12,20 @@ endif
# Your platform. See PLATS for possible values.
PLAT= none
+platform= $(shell uname)
+
CC= gcc
CFLAGS= -O3 -Wall -DLUA_COMPAT_ALL $(SYSCFLAGS) $(MYCFLAGS)
LDFLAGS= $(SYSLDFLAGS) $(MYLDFLAGS)
LIBS= -lm $(SYSLIBS) $(MYLIBS)
+ifeq ($(platform),Darwin)
+AR= /usr/bin/ar rc
+RANLIB= /usr/bin/ranlib
+else
AR= ar rc
RANLIB= ranlib
+endif
RM= rm -f
SYSCFLAGS=
diff --git a/proxmark3.sh b/proxmark3.sh
index b4cbd49fd..4cd6d76bc 100755
--- a/proxmark3.sh
+++ b/proxmark3.sh
@@ -3,54 +3,89 @@
FULLIMAGE="armsrc/obj/fullimage.elf"
BOOTIMAGE="bootrom/obj/bootrom.elf"
-cd $(dirname "$0")
+PM3PATH=$(dirname "$0")
+cd "$PM3PATH" || exit 1
function wait4proxmark_Linux {
echo >&2 "Waiting for Proxmark to appear..."
- while [ ! -c /dev/ttyACM? -a ! -c /dev/pm3-? ]; do
+ while true; do
+ PM3=$(find /dev/pm3-* /dev/ttyACM* 2>/dev/null | head -1)
+ if [[ $PM3 != "" ]]; then
+ break
+ fi
sleep .1
done
- local PM3=`ls -1 /dev/pm3-? /dev/ttyACM? 2>/dev/null | head -1`
- echo >&2 -e "Found proxmark on ${PM3}\n"
- echo $PM3
+ echo "$PM3"
}
function wait4proxmark_macOS {
echo >&2 "Waiting for Proxmark to appear..."
while true; do
- PM3=$(ls /dev/pm3-* /dev/cu.usbmodem* 2>/dev/null | head -1)
+ PM3=$(find /dev/pm3-* /dev/cu.usbmodem* 2>/dev/null | head -1)
if [[ $PM3 != "" ]]; then
- #echo >&2 -e "Found proxmark on $(ls /dev/pm3-* /dev/cu.usbmodem* 2>/dev/null | head -1)\n"
break
fi
sleep .1
done
- echo $PM3
+ echo "$PM3"
+}
+
+function wait4proxmark_Windows {
+ echo >&2 "Waiting for Proxmark to appear..."
+ while true; do
+ device=$(wmic path Win32_SerialPort where "PNPDeviceID like '%VID_9AC4&PID_4B8F%'" get DeviceID,PNPDeviceID 2>/dev/null | awk 'NR==2')
+ if [[ $device != "" ]]; then
+ PM3=${device/ */}
+ break
+ fi
+ sleep .1
+ done
+ echo "$PM3"
+}
+
+function wait4proxmark_WSL {
+ echo >&2 "Waiting for Proxmark to appear..."
+ while true; do
+ device=$(wmic.exe path Win32_SerialPort where "PNPDeviceID like '%VID_9AC4&PID_4B8F%'" get DeviceID,PNPDeviceID 2>/dev/null | awk 'NR==2')
+ if [[ $device != "" ]]; then
+ PM3=${device/ */}
+ PM3="/dev/ttyS${PM3#COM}"
+ break
+ fi
+ sleep .1
+ done
+ if [ -e "$PM3" ] && [ ! -w "$PM3" ]; then
+ echo "We need to give current user read/write access to $PM3"
+ sudo chmod 666 "$PM3"
+ fi
+ echo "$PM3"
}
SCRIPT=$(basename -- "$0")
if [ "$SCRIPT" = "proxmark3.sh" ]; then
- CMD=client/proxmark3
+ CMD() { client/proxmark3 "$@"; }
elif [ "$SCRIPT" = "flash-all.sh" ]; then
- CMD=client/flasher
- ARG1="-b $BOOTIMAGE"
- ARG2="$FULLIMAGE"
+ CMD() { client/flasher "$1" -b "$BOOTIMAGE" "$FULLIMAGE"; }
elif [ "$SCRIPT" = "flash-fullimage.sh" ]; then
- CMD=client/flasher
- ARG2="$FULLIMAGE"
+ CMD() { client/flasher "$1" "$FULLIMAGE"; }
elif [ "$SCRIPT" = "flash-bootrom.sh" ]; then
- CMD=client/flasher
- ARG1="-b $BOOTIMAGE"
+ CMD() { client/flasher "$1" -b "$BOOTIMAGE"; }
else
echo "Script ran under unknown name, abort: $SCRIPT"
exit 1
fi
HOSTOS=$(uname | awk '{print toupper($0)}')
if [ "$HOSTOS" = "LINUX" ]; then
- PORT=$(wait4proxmark_Linux)
+ if uname -a|grep -q Microsoft; then
+ PORT=$(wait4proxmark_WSL)
+ else
+ PORT=$(wait4proxmark_Linux)
+ fi
elif [ "$HOSTOS" = "DARWIN" ]; then
PORT=$(wait4proxmark_macOS)
+elif [[ "$HOSTOS" =~ MINGW(32|64)_NT* ]]; then
+ PORT=$(wait4proxmark_Windows)
else
echo "Host OS not recognized, abort: $HOSTOS"
exit 1
@@ -60,6 +95,5 @@ if [ "$PORT" = "" ]; then
exit 1
fi
-#echo Running "$CMD" "$PORT" $ARG1 $ARG2 "$@"
-"$CMD" "$PORT" $ARG1 $ARG2 "$@"
+CMD "$PORT" "$@"
exit $?
diff --git a/tools/jtag_openocd/general.cfg b/tools/jtag_openocd/general.cfg
new file mode 100644
index 000000000..6151d82bc
--- /dev/null
+++ b/tools/jtag_openocd/general.cfg
@@ -0,0 +1,3 @@
+# Ports
+telnet_port 4444
+gdb_port 3333
diff --git a/tools/jtag_openocd/interface-armusbocd.cfg b/tools/jtag_openocd/interface-armusbocd.cfg
index e4689e21b..4820136f0 100644
--- a/tools/jtag_openocd/interface-armusbocd.cfg
+++ b/tools/jtag_openocd/interface-armusbocd.cfg
@@ -1,7 +1,3 @@
-# Ports
-telnet_port 4444
-gdb_port 3333
-
# Commands specific to the Olimex ARM-USB-OCD Dongle
interface ft2232
ft2232_device_desc "Olimex OpenOCD JTAG"
diff --git a/tools/jtag_openocd/interface-busblaster.cfg b/tools/jtag_openocd/interface-busblaster.cfg
index 337e9a2de..bd1df2c5f 100644
--- a/tools/jtag_openocd/interface-busblaster.cfg
+++ b/tools/jtag_openocd/interface-busblaster.cfg
@@ -1,7 +1,3 @@
-# Ports
-telnet_port 4444
-gdb_port 3333
-
# Commands specific to the Bus Blaster
interface ftdi
ftdi_device_desc "Dual RS232-HS"
diff --git a/tools/jtag_openocd/interface-buspirate.cfg b/tools/jtag_openocd/interface-buspirate.cfg
index 4ed5b88a5..8eccadd79 100644
--- a/tools/jtag_openocd/interface-buspirate.cfg
+++ b/tools/jtag_openocd/interface-buspirate.cfg
@@ -1,7 +1,3 @@
-# Ports
-telnet_port 4444
-gdb_port 3333
-
# Commands specific to the BusPirate
interface buspirate
buspirate_port /dev/ttyUSB0
diff --git a/tools/jtag_openocd/interface-c232hm.cfg b/tools/jtag_openocd/interface-c232hm.cfg
new file mode 100644
index 000000000..f4d0f471a
--- /dev/null
+++ b/tools/jtag_openocd/interface-c232hm.cfg
@@ -0,0 +1,23 @@
+# Commands specific to the FTDI USB Hi-Speed to MPSSE Cable
+# http://www.ftdichip.com/Products/Cables/USBMPSSE.htm
+# C232HM-DDHSL-0 => 3.3V
+# C232HM-EDSL-0 => 5V !! don't apply 5V on the 3.3v pm3 pin !!
+
+# Pinouts:
+# Brown <> TMS
+# Yellow <> TDI
+# Green <> TDO
+# Orange <> TCK
+# Black <> GND
+# Red <> 3.3 (don't connect if C232HM-EDSL-0! power via USB instead)
+
+interface ftdi
+#ftdi_device_desc "C232HM-DDHSL-0"
+#ftdi_device_desc "C232HM-EDHSL-0"
+ftdi_vid_pid 0x0403 0x6014
+
+ftdi_layout_init 0x0008 0x400b
+ftdi_layout_signal LED -ndata 0x4000
+
+transport select jtag
+adapter_khz 1000
diff --git a/tools/jtag_openocd/interface-jlink.cfg b/tools/jtag_openocd/interface-jlink.cfg
index 56a04fef2..011cb7844 100644
--- a/tools/jtag_openocd/interface-jlink.cfg
+++ b/tools/jtag_openocd/interface-jlink.cfg
@@ -1,7 +1,3 @@
-# Ports
-telnet_port 4444
-gdb_port 3333
-
# Commands specific to the Segger J-Link
interface jlink
transport select jtag
diff --git a/tools/jtag_openocd/interface-jtagkey.cfg b/tools/jtag_openocd/interface-jtagkey.cfg
index 3e48c08df..420e6e97e 100644
--- a/tools/jtag_openocd/interface-jtagkey.cfg
+++ b/tools/jtag_openocd/interface-jtagkey.cfg
@@ -1,7 +1,3 @@
-# Ports
-telnet_port 4444
-gdb_port 3333
-
# Commands specific to the Amontec JTAGKey
interface ft2232
ft2232_device_desc "Amontec JTAGkey A"
diff --git a/tools/jtag_openocd/interface-raspberrypi.cfg b/tools/jtag_openocd/interface-raspberrypi.cfg
new file mode 100644
index 000000000..984afe41b
--- /dev/null
+++ b/tools/jtag_openocd/interface-raspberrypi.cfg
@@ -0,0 +1,37 @@
+# Config for using Raspberry Pi's expansion header
+# Using BCM2835 chip present in models Pi1, Pi2 and Pi Zero
+
+# Use short cables!
+# Make sure the CPU is not busy with other processes!
+# Connect pm3 before booting to avoid power loss & reboot problems
+# Pinouts:
+# 22 <> TMS
+# 19 <> TDI
+# 21 <> TDO
+# 23 <> TCK
+# 6 <> GND
+# 1 <> 3.3
+
+interface bcm2835gpio
+
+# This file is meant for first versions of Raspberry Pi
+# You can check yours with:
+# dd if=/proc/device-tree/soc/ranges bs=4 skip=1 count=1 2>/dev/null|xxd -p
+# if it returns 20000000, you're fine
+# if it returns 3F000000, use interface-raspberrypi2.cfg
+bcm2835gpio_peripheral_base 0x20000000
+
+# Transition delay calculation: SPEED_COEFF/khz - SPEED_OFFSET
+# These depend on system clock, calibrated for stock 700MHz
+# bcm2835gpio_speed SPEED_COEFF SPEED_OFFSET
+bcm2835gpio_speed_coeffs 113714 28
+
+# Each of the JTAG lines need a gpio number set: tck tms tdi tdo
+# Header pin numbers: 23 22 19 21
+bcm2835gpio_jtag_nums 11 25 10 9
+
+bcm2835gpio_srst_num 18
+reset_config srst_only srst_push_pull
+
+transport select jtag
+adapter_khz 1000
diff --git a/tools/jtag_openocd/interface-raspberrypi2.cfg b/tools/jtag_openocd/interface-raspberrypi2.cfg
new file mode 100644
index 000000000..b779cd290
--- /dev/null
+++ b/tools/jtag_openocd/interface-raspberrypi2.cfg
@@ -0,0 +1,37 @@
+# Config for using Raspberry Pi's expansion header
+# Using BCM2835 chip present in models 2+ and above
+
+# Use short cables!
+# Make sure the CPU is not busy with other processes!
+# Connect pm3 before booting to avoid power loss & reboot problems
+# Pinouts:
+# 22 <> TMS
+# 19 <> TDI
+# 21 <> TDO
+# 23 <> TCK
+# 6 <> GND
+# 1 <> 3.3
+
+interface bcm2835gpio
+
+# This file is meant for recent versions of Raspberry Pi
+# You can check yours with:
+# dd if=/proc/device-tree/soc/ranges bs=4 skip=1 count=1 2>/dev/null|xxd -p
+# if it returns 20000000, use interface-raspberrypi2.cfg
+# if it returns 3F000000, you're fine
+bcm2835gpio_peripheral_base 0x3F000000
+
+# Transition delay calculation: SPEED_COEFF/khz - SPEED_OFFSET
+# These depend on system clock, calibrated for stock 700MHz
+# bcm2835gpio_speed SPEED_COEFF SPEED_OFFSET
+bcm2835gpio_speed_coeffs 146203 36
+
+# Each of the JTAG lines need a gpio number set: tck tms tdi tdo
+# Header pin numbers: 23 22 19 21
+bcm2835gpio_jtag_nums 11 25 10 9
+
+bcm2835gpio_srst_num 18
+reset_config srst_only srst_push_pull
+
+transport select jtag
+adapter_khz 1000
diff --git a/tools/jtag_openocd/interface-shikra.cfg b/tools/jtag_openocd/interface-shikra.cfg
index 507c301b4..ba4b21419 100644
--- a/tools/jtag_openocd/interface-shikra.cfg
+++ b/tools/jtag_openocd/interface-shikra.cfg
@@ -1,7 +1,3 @@
-# Ports
-telnet_port 4444
-gdb_port 3333
-
# Commands specific to the Shikra
interface ftdi
transport select jtag
diff --git a/tools/jtag_openocd/interface-wiggler.cfg b/tools/jtag_openocd/interface-wiggler.cfg
index 88448de82..ce9a1da06 100644
--- a/tools/jtag_openocd/interface-wiggler.cfg
+++ b/tools/jtag_openocd/interface-wiggler.cfg
@@ -1,6 +1,3 @@
-telnet_port 4444
-gdb_port 3333
-
# Commands specific to the Wiggler
interface parport
parport_port 0x378
diff --git a/tools/jtag_openocd/openocd_configuration.sample b/tools/jtag_openocd/openocd_configuration.sample
new file mode 100644
index 000000000..466e28d9b
--- /dev/null
+++ b/tools/jtag_openocd/openocd_configuration.sample
@@ -0,0 +1,7 @@
+CONFIG_GEN=general.cfg
+CONFIG_CHIP=chip-at91sam7s.cfg
+IMAGE=../../recovery/proxmark3_recovery.bin
+DUMP="dump_$(date +'%Y%m%d-%H%M%S').bin"
+
+# Example using Segger Jlink:
+CONFIG_IF=interface-jlink.cfg
diff --git a/tools/jtag_openocd/openocd_flash_dump.sh b/tools/jtag_openocd/openocd_flash_dump.sh
new file mode 100755
index 000000000..497847dd1
--- /dev/null
+++ b/tools/jtag_openocd/openocd_flash_dump.sh
@@ -0,0 +1,9 @@
+#!/bin/bash
+
+. openocd_configuration || exit 1
+
+if [ -e "$DUMP" ]; then
+ echo "$DUMP exists already. Abort!"
+ exit 1
+fi
+openocd -f $CONFIG_GEN -f $CONFIG_IF -f $CONFIG_CHIP -c "init;halt;dump_image $DUMP 0x100000 0x80000;exit"
diff --git a/tools/jtag_openocd/openocd_flash_recovery.sh b/tools/jtag_openocd/openocd_flash_recovery.sh
index b51076d5a..2e88c52e6 100755
--- a/tools/jtag_openocd/openocd_flash_recovery.sh
+++ b/tools/jtag_openocd/openocd_flash_recovery.sh
@@ -1,12 +1,9 @@
#!/bin/bash
-# Example using Segger Jlink:
-CONFIG_CHIP=chip-at91sam7s.cfg
-CONFIG_IF=interface-jlink.cfg
-IMAGE=../../recovery/proxmark3_recovery.bin
+. openocd_configuration || exit 1
if [ ! -e "$IMAGE" ]; then
echo "$IMAGE missing. Abort!"
exit 1
fi
-openocd -f $CONFIG_IF -f $CONFIG_CHIP -c "init;halt;flash erase_sector 0 0 15;flash erase_sector 1 0 15;flash write_image $IMAGE 0x100000;exit"
+openocd -f $CONFIG_GEN -f $CONFIG_IF -f $CONFIG_CHIP -c "init;halt;flash erase_sector 0 0 15;flash erase_sector 1 0 15;flash write_image $IMAGE 0x100000;exit"
diff --git a/tools/jtag_openocd/openocd_interactive.sh b/tools/jtag_openocd/openocd_interactive.sh
new file mode 100755
index 000000000..ea7919da8
--- /dev/null
+++ b/tools/jtag_openocd/openocd_interactive.sh
@@ -0,0 +1,8 @@
+#!/bin/bash
+
+. openocd_configuration || exit 1
+
+echo "*********************************************"
+echo "Connect to OpenOCD via: telnet localhost $(awk '/^telnet_port/{print$2}' $CONFIG_GEN)"
+echo "*********************************************"
+openocd -f $CONFIG_GEN -f $CONFIG_IF -f $CONFIG_CHIP
diff --git a/uart/uart_posix.c b/uart/uart_posix.c
index 93b7229ce..ea535fd73 100644
--- a/uart/uart_posix.c
+++ b/uart/uart_posix.c
@@ -238,7 +238,8 @@ void uart_close(const serial_port sp) {
// Does the system allows us to place a lock on this file descriptor
int err = fcntl(spu->fd, F_SETLK, &fl);
if (err == -1) {
- //perror("fcntl");
+ //silent error message as it can be called from uart_open failing modes, e.g. when waiting for port to appear
+ //printf("[!] UART error while closing port\n");
}
close(spu->fd);
free(sp);